83.删除排序链表中的重复元素、108.将有序数组转换为二叉搜索树、181.超过经理收入的员工、182.查找重复的电子邮箱
This commit is contained in:
parent
4b5520d210
commit
bf3fadba8e
|
@ -39,6 +39,7 @@ mod q0070;
|
|||
mod q0078;
|
||||
mod q0080;
|
||||
mod q0081;
|
||||
mod q0083;
|
||||
mod q0086;
|
||||
mod q0087;
|
||||
mod q0088;
|
||||
|
@ -49,6 +50,7 @@ mod q0100;
|
|||
mod q0101;
|
||||
mod q0102;
|
||||
mod q0104;
|
||||
mod q0108;
|
||||
mod q0112;
|
||||
mod q0110;
|
||||
mod q0111;
|
||||
|
|
|
@ -0,0 +1,65 @@
|
|||
use crate::Solution;
|
||||
use crate::structure::ListNode;
|
||||
|
||||
impl Solution {
|
||||
/// [83.删除排序链表中的重复元素](https://leetcode-cn.com/problems/remove-duplicates-from-sorted-list/)
|
||||
///
|
||||
/// 2022-08-10 21:10:32
|
||||
///
|
||||
/// 给定一个已排序的链表的头 `head` , _删除所有重复的元素,使每个元素只出现一次_ 。返回 _已排序的链表_ 。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + 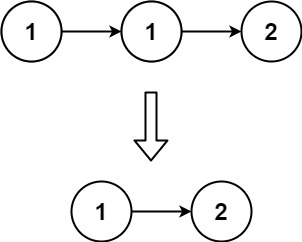```
|
||||
/// + **输入:**head = \[1,1,2\]
|
||||
/// + **输出:**\[1,2\]
|
||||
/// + **示例 2:**
|
||||
/// + 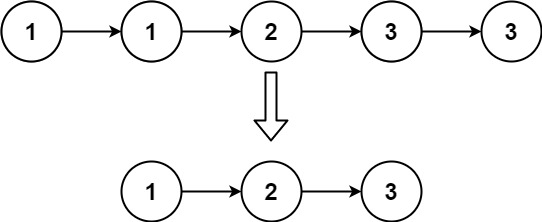```
|
||||
/// + **输入:**head = \[1,1,2,3,3\]
|
||||
/// + **输出:**\[1,2,3\]
|
||||
/// + **提示:**
|
||||
/// * 链表中节点数目在范围 `[0, 300]` 内
|
||||
/// * `-100 <= Node.val <= 100`
|
||||
/// * 题目数据保证链表已经按升序 **排列**
|
||||
/// + Related Topics
|
||||
/// * 链表
|
||||
pub fn delete_duplicates(mut head: Option<Box<ListNode>>) -> Option<Box<ListNode>> {
|
||||
/*let mut vec = Vec::<i32>::new();
|
||||
while let Some(n) = head {
|
||||
if Some(&n.val) != vec.last() {
|
||||
vec.push(n.val);
|
||||
}
|
||||
head = n.next;
|
||||
}
|
||||
let mut node = None;
|
||||
for val in vec.into_iter().rev() {
|
||||
node = Some(Box::new(ListNode { val, next: node }));
|
||||
}
|
||||
node*/
|
||||
if head.is_none() {
|
||||
return None
|
||||
}
|
||||
let mut node = head.as_mut().unwrap();
|
||||
let mut pre = node.val;
|
||||
while let Some(mut next) = node.next.take() {
|
||||
if pre == next.val { //去掉当前节点
|
||||
node.next = next.next;
|
||||
} else { // 还原
|
||||
pre = next.val;
|
||||
node.next = Some(next);
|
||||
node = node.next.as_mut().unwrap();
|
||||
}
|
||||
}
|
||||
head
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
use crate::structure::ListNode;
|
||||
|
||||
#[test]
|
||||
fn test_q0083() {
|
||||
assert_eq!(Solution::delete_duplicates(Some(Box::new(ListNode { val: 1, next: Some(Box::new(ListNode { val: 1, next: Some(Box::new(ListNode { val: 2, next: None })) })) }))), Some(Box::new(ListNode { val: 1, next: Some(Box::new(ListNode { val: 2, next: None })) })));
|
||||
}
|
||||
}
|
|
@ -0,0 +1,54 @@
|
|||
use crate::Solution;
|
||||
use crate::structure::TreeNode;
|
||||
|
||||
use std::rc::Rc;
|
||||
use std::cell::RefCell;
|
||||
|
||||
impl Solution {
|
||||
/// [108.将有序数组转换为二叉搜索树](https://leetcode-cn.com/problems/convert-sorted-array-to-binary-search-tree/)
|
||||
///
|
||||
/// 2022-08-10 21:43:44
|
||||
///
|
||||
/// 给你一个整数数组 `nums` ,其中元素已经按 **升序** 排列,请你将其转换为一棵 **高度平衡** 二叉搜索树。
|
||||
///
|
||||
/// **高度平衡** 二叉树是一棵满足「每个节点的左右两个子树的高度差的绝对值不超过 1 」的二叉树。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + 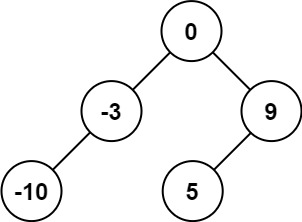
|
||||
/// + **输入:** nums = \[-10,-3,0,5,9\]
|
||||
/// + **输出:** \[0,-3,9,-10,null,5\]
|
||||
/// + **解释:** \[0,-10,5,null,-3,null,9\] 也将被视为正确答案:
|
||||
/// + 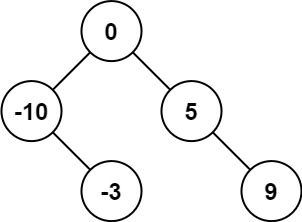
|
||||
/// + **示例 2:**
|
||||
/// + 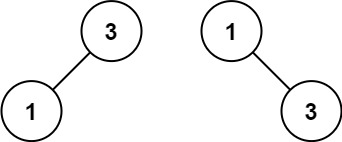
|
||||
/// + **输入:** nums = \[1,3\]
|
||||
/// + **输出:** \[3,1\]
|
||||
/// + **解释:** \[1,null,3\] 和 \[3,1\] 都是高度平衡二叉搜索树。
|
||||
/// + **提示:**
|
||||
/// * `1 <= nums.length <= 104`
|
||||
/// * `-104 <= nums[i] <= 104`
|
||||
/// * `nums` 按 **严格递增** 顺序排列
|
||||
/// + Related Topics
|
||||
/// * 树
|
||||
/// * 二叉搜索树
|
||||
/// * 数组
|
||||
/// * 分治
|
||||
/// * 二叉树
|
||||
pub fn sorted_array_to_bst(nums: Vec<i32>) -> Option<Rc<RefCell<TreeNode>>> {
|
||||
match nums.len() {
|
||||
0 => None,
|
||||
l => Some(Rc::new(RefCell::new(TreeNode { val: nums[l / 2], left: Self::sorted_array_to_bst(nums[..l / 2].to_vec()), right: Self::sorted_array_to_bst(nums[l / 2 + 1..].to_vec()) })))
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_q0108() {
|
||||
println!("{:?}", Solution::sorted_array_to_bst(vec![1, 3]));
|
||||
println!("{:?}", Solution::sorted_array_to_bst(vec![-10, -3, 0, 5, 9]));
|
||||
}
|
||||
}
|
|
@ -0,0 +1,114 @@
|
|||
# [181.超过经理收入的员工](https://leetcode-cn.com/problems/employees-earning-more-than-their-managers/)
|
||||
|
||||
2022-08-10 22:05:28
|
||||
|
||||
## SQL架构
|
||||
|
||||
```mysql
|
||||
Create table If Not Exists Employee
|
||||
(
|
||||
id int,
|
||||
name varchar(255),
|
||||
salary int,
|
||||
managerId int
|
||||
);
|
||||
Truncate table Employee;
|
||||
|
||||
insert into Employee (id, name, salary, managerId)
|
||||
values ('1', 'Joe', '70000', '3');
|
||||
|
||||
insert into Employee (id, name, salary, managerId)
|
||||
values ('2', 'Henry', '80000', '4');
|
||||
|
||||
insert into Employee (id, name, salary, managerId)
|
||||
values ('3', 'Sam', '60000', 'None');
|
||||
|
||||
insert into Employee (id, name, salary, managerId)
|
||||
values ('4', 'Max', '90000', 'None');
|
||||
```
|
||||
|
||||
## 题
|
||||
|
||||
表:`Employee`
|
||||
|
||||
```
|
||||
+-------------+---------+
|
||||
| Column Name | Type |
|
||||
+-------------+---------+
|
||||
| id | int |
|
||||
| name | varchar |
|
||||
| salary | int |
|
||||
| managerId | int |
|
||||
+-------------+---------+
|
||||
Id是该表的主键。
|
||||
该表的每一行都表示雇员的ID、姓名、工资和经理的ID。
|
||||
|
||||
```
|
||||
|
||||
编写一个SQL查询来查找收入比经理高的员工。
|
||||
|
||||
以 **任意顺序** 返回结果表。
|
||||
|
||||
查询结果格式如下所示。
|
||||
|
||||
**示例 1:**
|
||||
|
||||
```
|
||||
**输入:**
|
||||
Employee 表:
|
||||
+----+-------+--------+-----------+
|
||||
| id | name | salary | managerId |
|
||||
+----+-------+--------+-----------+
|
||||
| 1 | Joe | 70000 | 3 |
|
||||
| 2 | Henry | 80000 | 4 |
|
||||
| 3 | Sam | 60000 | Null |
|
||||
| 4 | Max | 90000 | Null |
|
||||
+----+-------+--------+-----------+
|
||||
**输出:**
|
||||
+----------+
|
||||
| Employee |
|
||||
+----------+
|
||||
| Joe |
|
||||
+----------+
|
||||
**解释:** Joe 是唯一挣得比经理多的雇员。
|
||||
```
|
||||
|
||||
Related Topics
|
||||
|
||||
* 数据库
|
||||
|
||||
## 解
|
||||
|
||||
```mysql
|
||||
SELECT a.Name AS 'Employee'
|
||||
FROM Employee AS a,
|
||||
Employee AS b
|
||||
WHERE a.ManagerId = b.Id
|
||||
AND a.Salary > b.Salary;
|
||||
```
|
||||
|
||||
```mysql
|
||||
SELECT a.Name AS 'Employee'
|
||||
FROM Employee a,
|
||||
Employee b
|
||||
WHERE a.ManagerId = b.Id
|
||||
AND a.Salary > b.Salary;
|
||||
```
|
||||
|
||||
```mysql
|
||||
SELECT a.NAME AS Employee
|
||||
FROM Employee AS a
|
||||
JOIN Employee AS b
|
||||
ON a.ManagerId = b.Id
|
||||
AND a.Salary > b.Salary;
|
||||
```
|
||||
|
||||
```mysql
|
||||
SELECT a.NAME AS Employee
|
||||
FROM Employee a
|
||||
JOIN Employee b
|
||||
ON a.ManagerId = b.Id
|
||||
AND a.Salary > b.Salary;
|
||||
```
|
||||
|
||||
不加`AS`的快一点好像。
|
|
@ -0,0 +1,74 @@
|
|||
# [182.查找重复的电子邮箱](https://leetcode-cn.com/problems/duplicate-emails/)
|
||||
|
||||
2022-08-10 22:24:58
|
||||
|
||||
## SQL架构
|
||||
|
||||
```mysql
|
||||
Create table If Not Exists Person
|
||||
(
|
||||
id int,
|
||||
email varchar(255)
|
||||
);
|
||||
Truncate table Person;
|
||||
|
||||
insert into Person (id, email)
|
||||
values ('1', 'a@b.com');
|
||||
|
||||
insert into Person (id, email)
|
||||
values ('2', 'c@d.com');
|
||||
|
||||
insert into Person (id, email)
|
||||
values ('3', 'a@b.com');
|
||||
```
|
||||
|
||||
## 题目
|
||||
|
||||
编写一个 SQL 查询,查找 `Person` 表中所有重复的电子邮箱。
|
||||
|
||||
**示例:**
|
||||
|
||||
```
|
||||
+----+---------+
|
||||
| Id | Email |
|
||||
+----+---------+
|
||||
| 1 | a@b.com |
|
||||
| 2 | c@d.com |
|
||||
| 3 | a@b.com |
|
||||
+----+---------+
|
||||
|
||||
```
|
||||
|
||||
根据以上输入,你的查询应返回以下结果:
|
||||
|
||||
```
|
||||
+---------+
|
||||
| Email |
|
||||
+---------+
|
||||
| a@b.com |
|
||||
+---------+
|
||||
|
||||
```
|
||||
|
||||
**说明:**所有电子邮箱都是小写字母。
|
||||
|
||||
Related Topics
|
||||
|
||||
* 数据库
|
||||
|
||||
## 解
|
||||
|
||||
```mysql
|
||||
select Email
|
||||
from (select Email, count(Email) as num
|
||||
from Person
|
||||
group by Email) as statistic
|
||||
where num > 1;
|
||||
```
|
||||
|
||||
```mysql
|
||||
select Email
|
||||
from Person
|
||||
group by Email
|
||||
having count(Email) > 1;
|
||||
```
|
Loading…
Reference in New Issue