66 lines
2.3 KiB
Rust
66 lines
2.3 KiB
Rust
use crate::Solution;
|
||
use crate::structure::ListNode;
|
||
|
||
impl Solution {
|
||
/// [83.删除排序链表中的重复元素](https://leetcode-cn.com/problems/remove-duplicates-from-sorted-list/)
|
||
///
|
||
/// 2022-08-10 21:10:32
|
||
///
|
||
/// 给定一个已排序的链表的头 `head` , _删除所有重复的元素,使每个元素只出现一次_ 。返回 _已排序的链表_ 。
|
||
///
|
||
/// + **示例 1:**
|
||
/// + 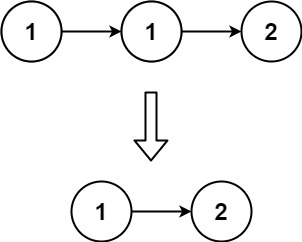```
|
||
/// + **输入:**head = \[1,1,2\]
|
||
/// + **输出:**\[1,2\]
|
||
/// + **示例 2:**
|
||
/// + 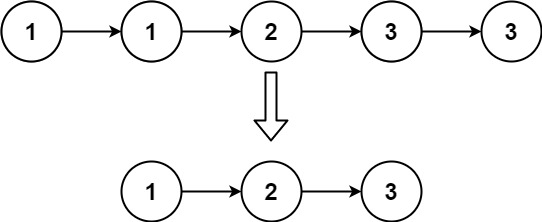```
|
||
/// + **输入:**head = \[1,1,2,3,3\]
|
||
/// + **输出:**\[1,2,3\]
|
||
/// + **提示:**
|
||
/// * 链表中节点数目在范围 `[0, 300]` 内
|
||
/// * `-100 <= Node.val <= 100`
|
||
/// * 题目数据保证链表已经按升序 **排列**
|
||
/// + Related Topics
|
||
/// * 链表
|
||
pub fn delete_duplicates(mut head: Option<Box<ListNode>>) -> Option<Box<ListNode>> {
|
||
/*let mut vec = Vec::<i32>::new();
|
||
while let Some(n) = head {
|
||
if Some(&n.val) != vec.last() {
|
||
vec.push(n.val);
|
||
}
|
||
head = n.next;
|
||
}
|
||
let mut node = None;
|
||
for val in vec.into_iter().rev() {
|
||
node = Some(Box::new(ListNode { val, next: node }));
|
||
}
|
||
node*/
|
||
if head.is_none() {
|
||
return None
|
||
}
|
||
let mut node = head.as_mut().unwrap();
|
||
let mut pre = node.val;
|
||
while let Some(mut next) = node.next.take() {
|
||
if pre == next.val { //去掉当前节点
|
||
node.next = next.next;
|
||
} else { // 还原
|
||
pre = next.val;
|
||
node.next = Some(next);
|
||
node = node.next.as_mut().unwrap();
|
||
}
|
||
}
|
||
head
|
||
}
|
||
}
|
||
|
||
#[cfg(test)]
|
||
mod test {
|
||
use crate::Solution;
|
||
use crate::structure::ListNode;
|
||
|
||
#[test]
|
||
fn test_q0083() {
|
||
assert_eq!(Solution::delete_duplicates(Some(Box::new(ListNode { val: 1, next: Some(Box::new(ListNode { val: 1, next: Some(Box::new(ListNode { val: 2, next: None })) })) }))), Some(Box::new(ListNode { val: 1, next: Some(Box::new(ListNode { val: 2, next: None })) })));
|
||
}
|
||
}
|