55 lines
2.1 KiB
Rust
55 lines
2.1 KiB
Rust
use crate::Solution;
|
||
use crate::structure::TreeNode;
|
||
|
||
use std::rc::Rc;
|
||
use std::cell::RefCell;
|
||
|
||
impl Solution {
|
||
/// [108.将有序数组转换为二叉搜索树](https://leetcode-cn.com/problems/convert-sorted-array-to-binary-search-tree/)
|
||
///
|
||
/// 2022-08-10 21:43:44
|
||
///
|
||
/// 给你一个整数数组 `nums` ,其中元素已经按 **升序** 排列,请你将其转换为一棵 **高度平衡** 二叉搜索树。
|
||
///
|
||
/// **高度平衡** 二叉树是一棵满足「每个节点的左右两个子树的高度差的绝对值不超过 1 」的二叉树。
|
||
///
|
||
/// + **示例 1:**
|
||
/// + 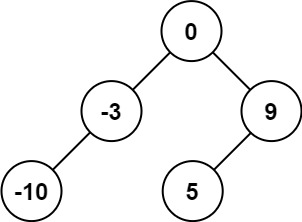
|
||
/// + **输入:** nums = \[-10,-3,0,5,9\]
|
||
/// + **输出:** \[0,-3,9,-10,null,5\]
|
||
/// + **解释:** \[0,-10,5,null,-3,null,9\] 也将被视为正确答案:
|
||
/// + 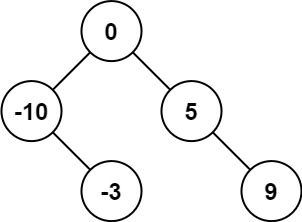
|
||
/// + **示例 2:**
|
||
/// + 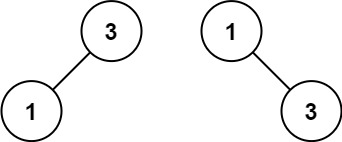
|
||
/// + **输入:** nums = \[1,3\]
|
||
/// + **输出:** \[3,1\]
|
||
/// + **解释:** \[1,null,3\] 和 \[3,1\] 都是高度平衡二叉搜索树。
|
||
/// + **提示:**
|
||
/// * `1 <= nums.length <= 104`
|
||
/// * `-104 <= nums[i] <= 104`
|
||
/// * `nums` 按 **严格递增** 顺序排列
|
||
/// + Related Topics
|
||
/// * 树
|
||
/// * 二叉搜索树
|
||
/// * 数组
|
||
/// * 分治
|
||
/// * 二叉树
|
||
pub fn sorted_array_to_bst(nums: Vec<i32>) -> Option<Rc<RefCell<TreeNode>>> {
|
||
match nums.len() {
|
||
0 => None,
|
||
l => Some(Rc::new(RefCell::new(TreeNode { val: nums[l / 2], left: Self::sorted_array_to_bst(nums[..l / 2].to_vec()), right: Self::sorted_array_to_bst(nums[l / 2 + 1..].to_vec()) })))
|
||
}
|
||
}
|
||
}
|
||
|
||
#[cfg(test)]
|
||
mod test {
|
||
use crate::Solution;
|
||
|
||
#[test]
|
||
fn test_q0108() {
|
||
println!("{:?}", Solution::sorted_array_to_bst(vec![1, 3]));
|
||
println!("{:?}", Solution::sorted_array_to_bst(vec![-10, -3, 0, 5, 9]));
|
||
}
|
||
}
|