623.在二叉树中增加一行
This commit is contained in:
parent
cae5428c7a
commit
d448d45f07
|
@ -125,6 +125,7 @@ mod q0575;
|
|||
mod q0598;
|
||||
mod q0605;
|
||||
mod q0617;
|
||||
mod q0623;
|
||||
mod q0628;
|
||||
mod q0633;
|
||||
mod q0643;
|
||||
|
|
|
@ -0,0 +1,138 @@
|
|||
use crate::Solution;
|
||||
use std::rc::Rc;
|
||||
use std::cell::RefCell;
|
||||
use std::cmp::Ordering;
|
||||
use crate::structure::TreeNode;
|
||||
|
||||
impl Solution {
|
||||
/// [623.在二叉树中增加一行](https://leetcode.cn/problems/add-one-row-to-tree/)
|
||||
///
|
||||
/// 2022-08-05 15:51:39
|
||||
///
|
||||
/// 给定一个二叉树的根 `root` 和两个整数 `val` 和 `depth` ,在给定的深度 `depth` 处添加一个值为 `val` 的节点行。
|
||||
///
|
||||
/// 注意,根节点 `root` 位于深度 `1` 。
|
||||
///
|
||||
/// + 加法规则如下:
|
||||
/// * 给定整数 `depth`,对于深度为 `depth - 1` 的每个非空树节点 `cur` ,创建两个值为 `val` 的树节点作为 `cur` 的左子树根和右子树根。
|
||||
/// * `cur` 原来的左子树应该是新的左子树根的左子树。
|
||||
/// * `cur` 原来的右子树应该是新的右子树根的右子树。
|
||||
/// * 如果 `depth == 1` 意味着 `depth - 1` 根本没有深度,那么创建一个树节点,值 `val` 作为整个原始树的新根,而原始树就是新根的左子树。
|
||||
/// + **示例 1:**
|
||||
/// + 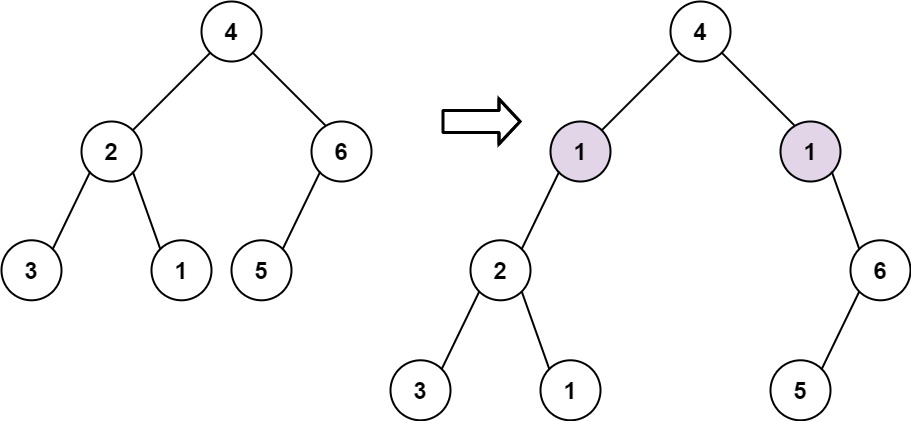
|
||||
/// + **输入:** root = \[4,2,6,3,1,5\], val = 1, depth = 2
|
||||
/// + **输出:** \[4,1,1,2,null,null,6,3,1,5\]
|
||||
/// + **示例 2:**
|
||||
/// + 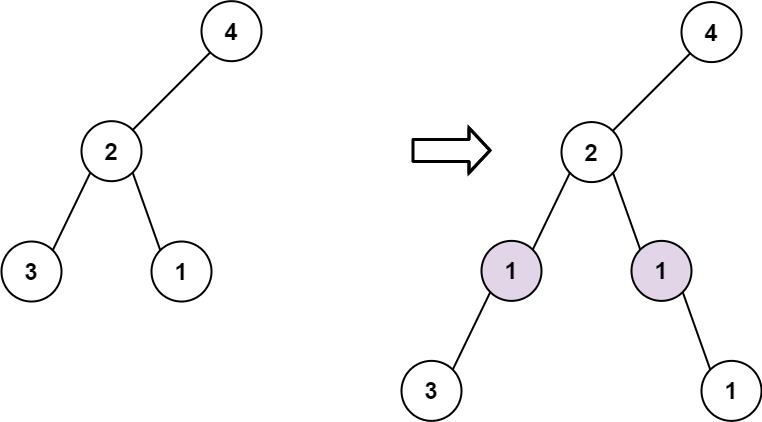
|
||||
/// + **输入:** root = \[4,2,null,3,1\], val = 1, depth = 3
|
||||
/// + **输出:** \[4,2,null,1,1,3,null,null,1\]
|
||||
/// + **提示:**
|
||||
/// * 节点数在 `[1, 104]` 范围内
|
||||
/// * 树的深度在 `[1, 104]`范围内
|
||||
/// * `-100 <= Node.val <= 100`
|
||||
/// * `-105 <= val <= 105`
|
||||
/// * `1 <= depth <= the depth of tree + 1`
|
||||
/// + Related Topics
|
||||
/// * 树
|
||||
/// * 深度优先搜索
|
||||
/// * 广度优先搜索
|
||||
/// * 二叉树
|
||||
/// * 👍 167
|
||||
/// * 👎 0
|
||||
pub fn add_one_row(root: Option<Rc<RefCell<TreeNode>>>, val: i32, depth: i32) -> Option<Rc<RefCell<TreeNode>>> {
|
||||
fn add_one_row_recursion(mut node: Option<Rc<RefCell<TreeNode>>>, curr_depth: i32, val: i32, depth: i32) -> Option<Rc<RefCell<TreeNode>>> {
|
||||
match curr_depth.cmp(&depth) {
|
||||
Ordering::Less => match node {
|
||||
Some(mut n) => match n.borrow_mut() {
|
||||
mut p => Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: p.val,
|
||||
left: add_one_row_recursion(p.left.take(), curr_depth + 1, val, depth),
|
||||
right: add_one_row_recursion(p.right.take(), curr_depth + 1, val, depth),
|
||||
})))
|
||||
},
|
||||
_ => None
|
||||
}
|
||||
Ordering::Equal => match node {
|
||||
Some(mut n) => match n.borrow_mut() {
|
||||
mut p => Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: p.val,
|
||||
left: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val,
|
||||
left: p.left.take(),
|
||||
right: None,
|
||||
}))),
|
||||
right: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val,
|
||||
left: None,
|
||||
right: p.right.take(),
|
||||
}))),
|
||||
})))
|
||||
},
|
||||
_ => None
|
||||
}
|
||||
_ => Some(Rc::new(RefCell::new(TreeNode {
|
||||
val,
|
||||
left: node,
|
||||
right: None,
|
||||
})))
|
||||
}
|
||||
}
|
||||
add_one_row_recursion(root, 1, val, depth - 1)
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
use std::rc::Rc;
|
||||
use std::cell::RefCell;
|
||||
use crate::structure::TreeNode;
|
||||
|
||||
#[test]
|
||||
fn test_q0623() {
|
||||
let tree = Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 4,
|
||||
left: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 2,
|
||||
left: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 3,
|
||||
left: None,
|
||||
right: None,
|
||||
}))),
|
||||
right: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 1,
|
||||
left: None,
|
||||
right: None,
|
||||
}))),
|
||||
}))),
|
||||
right: None,
|
||||
})));
|
||||
let ans = Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 4,
|
||||
left: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 2,
|
||||
left: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 1,
|
||||
left: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 3,
|
||||
left: None,
|
||||
right: None,
|
||||
}))),
|
||||
right: None,
|
||||
}))),
|
||||
right: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 1,
|
||||
left: None,
|
||||
right: Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 1,
|
||||
left: None,
|
||||
right: None,
|
||||
}))),
|
||||
}))),
|
||||
}))),
|
||||
right: None,
|
||||
})));
|
||||
assert_eq!(Solution::add_one_row(tree, 1, 3), ans);
|
||||
}
|
||||
}
|
Loading…
Reference in New Issue