112.路径总和
This commit is contained in:
parent
0d485bb0e9
commit
828c769814
|
@ -25,6 +25,7 @@ mod q0094;
|
|||
mod q0100;
|
||||
mod q0101;
|
||||
mod q0102;
|
||||
mod q0112;
|
||||
mod q0110;
|
||||
mod q0111;
|
||||
mod q0118;
|
||||
|
|
|
@ -0,0 +1,70 @@
|
|||
use crate::Solution;
|
||||
use crate::structure::TreeNode;
|
||||
use std::rc::Rc;
|
||||
use std::cell::RefCell;
|
||||
|
||||
impl Solution {
|
||||
/// [112.路径总和](https://leetcode-cn.com/problems/path-sum/)
|
||||
///
|
||||
/// 2022-02-10 17:27:58
|
||||
/// 给你二叉树的根节点 `root` 和一个表示目标和的整数 `targetSum` 。判断该树中是否存在 **根节点到叶子节点** 的路径,这条路径上所有节点值相加等于目标和 `targetSum` 。如果存在,返回 `true` ;否则,返回 `false` 。
|
||||
///
|
||||
/// + **叶子节点** 是指没有子节点的节点。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + 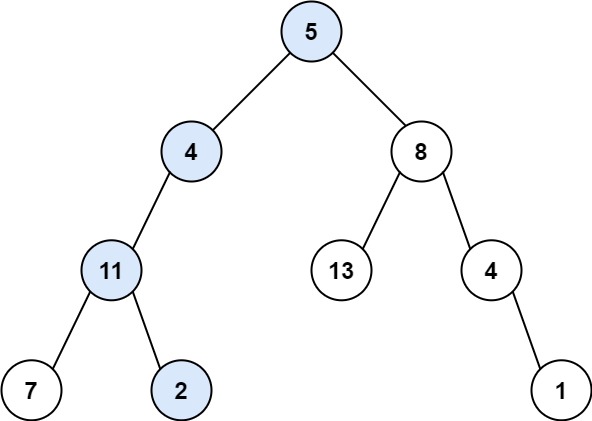
|
||||
/// + **输入:**root = \[5,4,8,11,null,13,4,7,2,null,null,null,1\], targetSum = 22
|
||||
/// + **输出:**true
|
||||
/// + **解释:**等于目标和的根节点到叶节点路径如上图所示。
|
||||
/// + **示例 2:**
|
||||
/// + 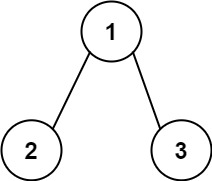
|
||||
/// + **输入:**root = \[1,2,3\], targetSum = 5
|
||||
/// + **输出:**false
|
||||
/// + **解释:**树中存在两条根节点到叶子节点的路径:
|
||||
/// - (1 --> 2): 和为 3
|
||||
/// - (1 --> 3): 和为 4
|
||||
/// - 不存在 sum = 5 的根节点到叶子节点的路径。
|
||||
/// + **示例 3:**
|
||||
/// + **输入:**root = \[\], targetSum = 0
|
||||
/// + **输出:**false
|
||||
/// + **解释:**由于树是空的,所以不存在根节点到叶子节点的路径。
|
||||
/// + **提示:**
|
||||
/// * 树中节点的数目在范围 `[0, 5000]` 内
|
||||
/// * `-1000 <= Node.val <= 1000`
|
||||
/// * `-1000 <= targetSum <= 1000`
|
||||
/// + Related Topics
|
||||
/// * 树
|
||||
/// * 深度优先搜索
|
||||
/// * 广度优先搜索
|
||||
/// * 二叉树
|
||||
/// * 👍 780
|
||||
/// * 👎 0
|
||||
pub fn has_path_sum(root: Option<Rc<RefCell<TreeNode>>>, target_sum: i32) -> bool {
|
||||
match root {
|
||||
Some(node) => {
|
||||
let mut p = node.borrow_mut();
|
||||
(p.left.is_none() && p.right.is_none() && p.val == target_sum) || Self::has_path_sum(p.left.take(), target_sum - p.val) || Self::has_path_sum(p.right.take(), target_sum - p.val)
|
||||
}
|
||||
_ => false
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
use crate::structure::TreeNode;
|
||||
use std::rc::Rc;
|
||||
use std::cell::RefCell;
|
||||
|
||||
#[test]
|
||||
fn test_q0112() {
|
||||
let tree = Some(Rc::new(RefCell::new(TreeNode {
|
||||
val: 1,
|
||||
left: Some(Rc::new(RefCell::new(TreeNode::new(2)))),
|
||||
right: Some(Rc::new(RefCell::new(TreeNode::new(4)))),
|
||||
})));
|
||||
assert_eq!(Solution::has_path_sum(tree, 6), false);
|
||||
}
|
||||
}
|
Loading…
Reference in New Issue