迁移实现
This commit is contained in:
parent
cbc454169c
commit
80aae26c97
|
@ -0,0 +1,37 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [面试题 05.01.插入](https://leetcode.cn/problems/insert-into-bits-lcci/)
|
||||
///
|
||||
/// 2022-08-09 10:41:48
|
||||
///
|
||||
/// 给定两个整型数字 `N` 与 `M`,以及表示比特位置的 `i` 与 `j`(`i <= j`,且从 0 位开始计算)。
|
||||
///
|
||||
/// 编写一种方法,使 `M` 对应的二进制数字插入 `N` 对应的二进制数字的第 `i ~ j` 位区域,不足之处用 `0` 补齐。具体插入过程如图所示。
|
||||
///
|
||||
/// 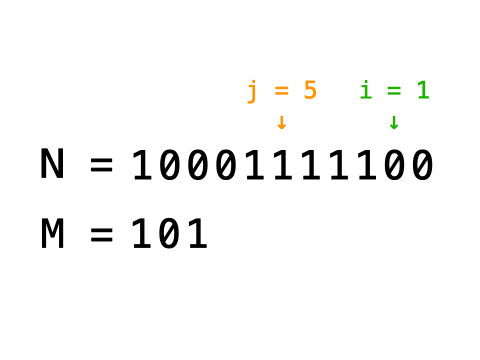
|
||||
///
|
||||
/// 题目保证从 `i` 位到 `j` 位足以容纳 `M`, 例如: `M = 10011`,则 `i~j` 区域至少可容纳 5 位。
|
||||
///
|
||||
/// + **示例1:**
|
||||
/// + **输入**:N = 1024(10000000000), M = 19(10011), i = 2, j = 6
|
||||
/// + **输出**:N = 1100(10001001100)
|
||||
/// + **示例2:**
|
||||
/// + **输入**: N = 0, M = 31(11111), i = 0, j = 4
|
||||
/// + **输出**:N = 31(11111)
|
||||
/// + Related Topics
|
||||
/// * 位运算
|
||||
pub fn insert_bits(n: i32, m: i32, i: i32, j: i32) -> i32 {
|
||||
n & !(((1 << (j - i + 1)) - 1) << i) | (m << i)
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_m0501() {
|
||||
assert_eq!(Solution::insert_bits(1024, 19, 2, 6), 1100);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,41 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [面试题 08.03.魔术索引](https://leetcode.cn/problems/magic-index-lcci/)
|
||||
///
|
||||
/// 2022-08-09 10:18:13
|
||||
///
|
||||
/// 魔术索引。 在数组`A[0...n-1]`中,有所谓的魔术索引,满足条件`A[i] = i`。给定一个有序整数数组,编写一种方法找出魔术索引,若有的话,在数组A中找出一个魔术索引,如果没有,则返回-1。若有多个魔术索引,返回索引值最小的一个。
|
||||
///
|
||||
/// + **示例1:**
|
||||
/// + **输入**:nums = \[0, 2, 3, 4, 5\]
|
||||
/// + **输出**:0
|
||||
/// + **说明**: 0下标的元素为0
|
||||
/// + **示例2:**
|
||||
/// + **输入**:nums = \[1, 1, 1\]
|
||||
/// + **输出**:1
|
||||
/// + **说明:**
|
||||
/// 1. nums长度在\[1, 1000000\]之间
|
||||
/// 2. 此题为原书中的 Follow-up,即数组中可能包含重复元素的版本
|
||||
/// + Related Topics
|
||||
/// * 数组
|
||||
/// * 二分查找
|
||||
pub fn find_magic_index(nums: Vec<i32>) -> i32 {
|
||||
for (i, val) in nums.into_iter().enumerate() {
|
||||
if val as usize == i {
|
||||
return i as i32;
|
||||
}
|
||||
}
|
||||
-1
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_m0803() {
|
||||
assert_eq!(Solution::find_magic_index(vec![0, 2, 3, 4, 5]), 0);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,35 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [面试题 16.01.交换数字](https://leetcode.cn/problems/swap-numbers-lcci/)
|
||||
///
|
||||
/// 2022-08-09 10:12:25
|
||||
///
|
||||
/// 编写一个函数,不用临时变量,直接交换`numbers = [a, b]`中`a`与`b`的值。
|
||||
///
|
||||
/// + **示例:**
|
||||
/// + **输入:** numbers = \[1,2\]
|
||||
/// + **输出:** \[2,1\]
|
||||
/// + **提示:**
|
||||
/// * `numbers.length == 2`
|
||||
/// * `-2147483647 <= numbers[i] <= 2147483647`
|
||||
/// + Related Topics
|
||||
/// * 位运算
|
||||
/// * 数学
|
||||
pub fn swap_numbers(mut numbers: Vec<i32>) -> Vec<i32> {
|
||||
numbers[0] ^= numbers[1];
|
||||
numbers[1] ^= numbers[0];
|
||||
numbers[0] ^= numbers[1];
|
||||
numbers
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_m1601() {
|
||||
assert_eq!(Solution::swap_numbers(vec![1, 2]), vec![2, 1]);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,39 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [面试题 16.07.最大数值](https://leetcode.cn/problems/maximum-lcci/)
|
||||
///
|
||||
/// 2022-08-09 10:36:43
|
||||
///
|
||||
/// 编写一个方法,找出两个数字`a`和`b`中最大的那一个。不得使用if-else或其他比较运算符。
|
||||
///
|
||||
/// + **示例:**
|
||||
/// + **输入:** a = 1, b = 2
|
||||
/// + **输出:** 2
|
||||
/// + Related Topics
|
||||
/// * 位运算
|
||||
/// * 脑筋急转弯
|
||||
/// * 数学
|
||||
/// # 方法1
|
||||
/// 内置函数
|
||||
/// ```rust
|
||||
/// pub fn maximum(a: i32, b: i32) -> i32 {
|
||||
/// std::cmp::max(a, b)
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法2
|
||||
/// 数学公式 max(a, b) = (|a - b| + a + b) / 2。使用64位计算,避免溢出。实现见源码。
|
||||
pub fn maximum(a: i32, b: i32) -> i32 {
|
||||
((a as i64 - b as i64).abs() + a as i64 + b as i64 >> 1) as i32
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_m1607() {
|
||||
assert_eq!(Solution::maximum(1, 2), 2);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,32 @@
|
|||
use crate::Solution;
|
||||
|
||||
|
||||
impl Solution {
|
||||
/// [面试题 17.09.第 k 个数](https://leetcode.cn/problems/get-kth-magic-number-lcci/)
|
||||
///
|
||||
/// 2022-08-08 16:53:54
|
||||
///
|
||||
/// 有些数的素因子只有 3,5,7,请设计一个算法找出第 k 个数。注意,不是必须有这些素因子,而是必须不包含其他的素因子。例如,前几个数按顺序应该是 1,3,5,7,9,15,21。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + **输入:** k = 5
|
||||
/// + **输出:** 9
|
||||
/// + Related Topics
|
||||
/// * 哈希表
|
||||
/// * 数学
|
||||
/// * 动态规划
|
||||
/// * 堆(优先队列)
|
||||
pub fn get_kth_magic_number(k: i32) -> i32 {
|
||||
unimplemented!();
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_m1709() {
|
||||
unimplemented!()
|
||||
}
|
||||
}
|
|
@ -1,4 +1,9 @@
|
|||
mod j1064;
|
||||
mod m0501;
|
||||
mod m0803;
|
||||
mod m1601;
|
||||
mod m1607;
|
||||
mod m1709;
|
||||
mod m1721;
|
||||
mod q0001;
|
||||
mod q0002;
|
||||
|
@ -180,17 +185,23 @@ mod q1202;
|
|||
mod q1203;
|
||||
mod q1218;
|
||||
mod q1269;
|
||||
mod q1281;
|
||||
mod q1288;
|
||||
mod q1295;
|
||||
mod q1310;
|
||||
mod q1342;
|
||||
mod q1374;
|
||||
mod q1403;
|
||||
mod q1404;
|
||||
mod q1408;
|
||||
mod q1413;
|
||||
mod q1447;
|
||||
mod q1473;
|
||||
mod q1480;
|
||||
mod q1482;
|
||||
mod q1486;
|
||||
mod q1502;
|
||||
mod q1678;
|
||||
mod q1720;
|
||||
mod q1723;
|
||||
mod q1734;
|
||||
|
|
|
@ -0,0 +1,49 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [1281.整数的各位积和之差](https://leetcode.cn/problems/subtract-the-product-and-sum-of-digits-of-an-integer/)
|
||||
///
|
||||
/// 2022-08-09 12:28:53
|
||||
///
|
||||
/// 给你一个整数 `n`,请你帮忙计算并返回该整数「各位数字之积」与「各位数字之和」的差。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + **输入:** n = 234
|
||||
/// + **输出:** 15
|
||||
/// + **解释:**
|
||||
/// + 各位数之积 = 2 \* 3 \* 4 = 24
|
||||
/// + 各位数之和 = 2 + 3 + 4 = 9
|
||||
/// + 结果 = 24 - 9 = 15
|
||||
/// **示例 2:**
|
||||
/// + **输入:** n = 4421
|
||||
/// + **输出:** 21
|
||||
/// + **解释:**
|
||||
/// - 各位数之积 = 4 \* 4 \* 2 \* 1 = 32
|
||||
/// - 各位数之和 = 4 + 4 + 2 + 1 = 11
|
||||
/// - 结果 = 32 - 11 = 21
|
||||
/// + **提示:**
|
||||
/// * `1 <= n <= 10^5`
|
||||
/// + Related Topics
|
||||
/// * 数学
|
||||
pub fn subtract_product_and_sum(mut n: i32) -> i32 {
|
||||
let mut add = 0;
|
||||
let mut mul = 1;
|
||||
while n > 0 {
|
||||
let digit = n % 10;
|
||||
n /= 10;
|
||||
add += digit;
|
||||
mul *= digit;
|
||||
}
|
||||
mul - add
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_q1281() {
|
||||
assert_eq!(Solution::subtract_product_and_sum(234), 15);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,84 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [1295.统计位数为偶数的数字](https://leetcode.cn/problems/find-numbers-with-even-number-of-digits/)
|
||||
///
|
||||
/// 2022-08-09 12:34:55
|
||||
///
|
||||
/// 给你一个整数数组 `nums`,请你返回其中位数为 **偶数** 的数字的个数。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + **输入:** nums = \[12,345,2,6,7896\]
|
||||
/// + **输出:** 2
|
||||
/// + **解释:**
|
||||
/// - 12 是 2 位数字(位数为偶数)
|
||||
/// - 345 是 3 位数字(位数为奇数)
|
||||
/// - 2 是 1 位数字(位数为奇数)
|
||||
/// - 6 是 1 位数字 位数为奇数)
|
||||
/// - 7896 是 4 位数字(位数为偶数)
|
||||
/// - 因此只有 12 和 7896 是位数为偶数的数字
|
||||
/// + **示例 2:**
|
||||
/// + **输入:** nums = \[555,901,482,1771\]
|
||||
/// + **输出:** 1
|
||||
/// + **解释:**
|
||||
/// - 只有 1771 是位数为偶数的数字。
|
||||
/// + **提示:**
|
||||
/// * `1 <= nums.length <= 500`
|
||||
/// * `1 <= nums[i] <= 10^5`
|
||||
/// + Related Topics
|
||||
/// * 数组
|
||||
/// # 方法1 字符串长度
|
||||
/// ```rust
|
||||
/// pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
/// nums.iter().fold(0, |mut acc, x| {
|
||||
/// acc += (1usize - (x.to_string().len() & 1usize)) as i32;
|
||||
/// acc
|
||||
/// })
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法2 取以10为底的对数
|
||||
/// ```rust
|
||||
/// pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
/// nums.iter().map(|&x| { ((x as f32).log10()) as i32 & 1 }).sum()
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法3 除10
|
||||
/// ```rust
|
||||
/// pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
/// let mut res = 0;
|
||||
/// for mut num in nums {
|
||||
/// let mut b = 0;
|
||||
/// while num > 9 {
|
||||
/// num /= 10;
|
||||
/// b += 1;
|
||||
/// }
|
||||
/// res += b & 1;
|
||||
/// }
|
||||
/// res
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法4 暴力
|
||||
pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
/*nums.iter().fold(0i32, |acc, &x| acc + match x {
|
||||
10..=99 | 1000..=9999 | 100000 => 1,
|
||||
_ => 0
|
||||
})*/
|
||||
/*nums.into_iter().map(|a| match a {
|
||||
10..=99 | 1000..=9999 | 100000 => 1,
|
||||
_ => 0
|
||||
}).sum()*/
|
||||
//nums.into_iter().filter(|x| x.to_string().len() & 1 == 0).count() as i32
|
||||
//nums.into_iter().filter(|&x| (((x as f32).log10() as i32) & 1) == 1).count() as i32
|
||||
nums.into_iter().filter(|&x| 9 < x && x < 100 || 999 < x && x < 10000 || x == 100000).count() as i32
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_q1295() {
|
||||
assert_eq!(Solution::find_numbers(vec![12, 345, 2, 6, 7896]), 2);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,59 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [1342.将数字变成 0 的操作次数](https://leetcode.cn/problems/number-of-steps-to-reduce-a-number-to-zero/)
|
||||
///
|
||||
/// 2022-08-09 13:43:16
|
||||
///
|
||||
/// 给你一个非负整数 `num` ,请你返回将它变成 0 所需要的步数。 如果当前数字是偶数,你需要把它除以 2 ;否则,减去 1 。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + **输入:** num = 14
|
||||
/// + **输出:** 6
|
||||
/// + **解释:**
|
||||
/// + 步骤 1) 14 是偶数,除以 2 得到 7 。
|
||||
/// + 步骤 2) 7 是奇数,减 1 得到 6 。
|
||||
/// + 步骤 3) 6 是偶数,除以 2 得到 3 。
|
||||
/// + 步骤 4) 3 是奇数,减 1 得到 2 。
|
||||
/// + 步骤 5) 2 是偶数,除以 2 得到 1 。
|
||||
/// + 步骤 6) 1 是奇数,减 1 得到 0 。
|
||||
/// + **示例 2:**
|
||||
/// + **输入:** num = 8
|
||||
/// + **输出:** 4
|
||||
/// + **解释:**
|
||||
/// + 步骤 1) 8 是偶数,除以 2 得到 4 。
|
||||
/// + 步骤 2) 4 是偶数,除以 2 得到 2 。
|
||||
/// + 步骤 3) 2 是偶数,除以 2 得到 1 。
|
||||
/// + 步骤 4) 1 是奇数,减 1 得到 0 。
|
||||
/// + **示例 3:**
|
||||
/// + **输入:** num = 123
|
||||
/// + **输出:** 12
|
||||
/// + **提示:**
|
||||
/// * `0 <= num <= 10^6`
|
||||
/// + Related Topics
|
||||
/// * 位运算
|
||||
/// * 数学
|
||||
pub fn number_of_steps(mut num: i32) -> i32 {
|
||||
/*let mut res = 0;
|
||||
while num > 1 {
|
||||
res += 1 + (num & 1);
|
||||
num >>= 1;
|
||||
}
|
||||
res + num*/
|
||||
match num > 1 {
|
||||
true => 1 + (num & 1) + Self::number_of_steps(num >> 1),
|
||||
_ => num
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_q1342() {
|
||||
assert_eq!(Solution::number_of_steps(123), 12);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,80 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [1404.将二进制表示减到 1 的步骤数](https://leetcode.cn/problems/number-of-steps-to-reduce-a-number-in-binary-representation-to-one/)
|
||||
///
|
||||
/// 2022-08-09 10:57:36
|
||||
///
|
||||
/// 给你一个以二进制形式表示的数字 `s` 。请你返回按下述规则将其减少到 1 所需要的步骤数:
|
||||
///
|
||||
/// * 如果当前数字为偶数,则将其除以 2 。
|
||||
/// * 如果当前数字为奇数,则将其加上 1 。
|
||||
///
|
||||
/// 题目保证你总是可以按上述规则将测试用例变为 1 。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + **输入:** s = "1101"
|
||||
/// + **输出:** 6
|
||||
/// + **解释:** "1101" 表示十进制数 13 。
|
||||
/// - Step 1) 13 是奇数,加 1 得到 14
|
||||
/// - Step 2) 14 是偶数,除 2 得到 7
|
||||
/// - Step 3) 7 是奇数,加 1 得到 8
|
||||
/// - Step 4) 8 是偶数,除 2 得到 4
|
||||
/// - Step 5) 4 是偶数,除 2 得到 2
|
||||
/// - Step 6) 2 是偶数,除 2 得到 1
|
||||
/// + **示例 2:**
|
||||
/// + **输入:** s = "10"
|
||||
/// + **输出:** 1
|
||||
/// + **解释:** "10" 表示十进制数 2 。
|
||||
/// - Step 1) 2 是偶数,除 2 得到 1
|
||||
/// + **示例 3:**
|
||||
/// + **输入:** s = "1"
|
||||
/// + **输出:** 0
|
||||
/// + **提示:**
|
||||
/// * `1 <= s.length <= 500`
|
||||
/// * `s` 由字符 `'0'` 或 `'1'` 组成。
|
||||
/// * `s[0] == '1'`
|
||||
/// + Related Topics
|
||||
/// * 位运算
|
||||
/// * 字符串
|
||||
pub fn num_steps(s: String) -> i32 {
|
||||
let mut result = 0;
|
||||
let mut meet1 = false;
|
||||
|
||||
for (i, c) in s.chars().rev().enumerate() {
|
||||
match c {
|
||||
'0' => result += meet1.then(|| 2).unwrap_or(1),
|
||||
_ => match meet1 {
|
||||
true => result += 1,
|
||||
_ => {
|
||||
result += (i != (s.len() - 1)).then(|| 2).unwrap_or(0);
|
||||
meet1 = true;
|
||||
}
|
||||
}
|
||||
}
|
||||
/*if c == '0' {
|
||||
result += if meet1 { 2 } else { 1 }
|
||||
} else {
|
||||
if !meet1 {
|
||||
if i != (s.len() - 1) {
|
||||
result += 2;
|
||||
}
|
||||
meet1 = true;
|
||||
} else {
|
||||
result += 1;
|
||||
}
|
||||
}*/
|
||||
}
|
||||
result
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_q1404() {
|
||||
assert_eq!(Solution::num_steps("1101".to_owned()), 6);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,45 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [1480.一维数组的动态和](https://leetcode.cn/problems/running-sum-of-1d-array/)
|
||||
///
|
||||
/// 2022-08-09 11:20:52
|
||||
///
|
||||
/// 给你一个数组 `nums` 。数组「动态和」的计算公式为:`runningSum[i] = sum(nums[0]…nums[i])` 。
|
||||
///
|
||||
/// 请返回 `nums` 的动态和。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + **输入:** nums = \[1,2,3,4\]
|
||||
/// + **输出:** \[1,3,6,10\]
|
||||
/// + **解释:** 动态和计算过程为 \[1, 1+2, 1+2+3, 1+2+3+4\] 。
|
||||
/// + **示例 2:**
|
||||
/// + **输入:** nums = \[1,1,1,1,1\]
|
||||
/// + **输出:** \[1,2,3,4,5\]
|
||||
/// + **解释:** 动态和计算过程为 \[1, 1+1, 1+1+1, 1+1+1+1, 1+1+1+1+1\] 。
|
||||
/// + **示例 3:**
|
||||
/// + **输入:** nums = \[3,1,2,10,1\]
|
||||
/// + **输出:** \[3,4,6,16,17\]
|
||||
/// + **提示:**
|
||||
/// * `1 <= nums.length <= 1000`
|
||||
/// * `-10^6 <= nums[i] <= 10^6`
|
||||
/// + Related Topics
|
||||
/// * 数组
|
||||
/// * 前缀和
|
||||
pub fn running_sum(nums: Vec<i32>) -> Vec<i32> {
|
||||
nums.into_iter().scan(0, |s, i| {
|
||||
*s += i;
|
||||
Some(*s)
|
||||
}).collect()
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_q1480() {
|
||||
assert_eq!(Solution::running_sum(vec![1, 2, 3, 4]), vec![1, 3, 6, 10]);
|
||||
}
|
||||
}
|
|
@ -0,0 +1,60 @@
|
|||
use crate::Solution;
|
||||
|
||||
impl Solution {
|
||||
/// [1678.设计 Goal 解析器](https://leetcode.cn/problems/goal-parser-interpretation/)
|
||||
///
|
||||
/// 2022-08-09 14:02:12
|
||||
///
|
||||
/// 请你设计一个可以解释字符串 `command` 的 **Goal 解析器** 。`command` 由 `"G"`、`"()"` 和/或 `"(al)"` 按某种顺序组成。Goal 解析器会将 `"G"` 解释为字符串 `"G"`、`"()"` 解释为字符串 `"o"` ,`"(al)"` 解释为字符串 `"al"` 。然后,按原顺序将经解释得到的字符串连接成一个字符串。
|
||||
///
|
||||
/// 给你字符串 `command` ,返回 **Goal 解析器** 对 `command` 的解释结果。
|
||||
///
|
||||
/// + **示例 1:**
|
||||
/// + **输入:** command = "G()(al)"
|
||||
/// + **输出:** "Goal"
|
||||
/// + **解释:** Goal 解析器解释命令的步骤如下所示:
|
||||
/// - G -> G
|
||||
/// - () -> o
|
||||
/// - (al) -> al
|
||||
/// - 最后连接得到的结果是 "Goal"
|
||||
/// + **示例 2:**
|
||||
/// + **输入:** command = "G()()()()(al)"
|
||||
/// + **输出:** "Gooooal"
|
||||
/// + **示例 3:**
|
||||
/// + **输入:** command = "(al)G(al)()()G"
|
||||
/// + **输出:** "alGalooG"
|
||||
/// + **提示:**
|
||||
/// * `1 <= command.length <= 100`
|
||||
/// * `command` 由 `"G"`、`"()"` 和/或 `"(al)"` 按某种顺序组成
|
||||
/// + Related Topics
|
||||
/// * 字符串
|
||||
pub fn interpret(command: String) -> String {
|
||||
let mut answer = vec![];
|
||||
let mut pre = b'\0';
|
||||
for ch in command.into_bytes() {
|
||||
if ch == b'G' {
|
||||
answer.push(ch);
|
||||
} else if ch == b')' {
|
||||
if pre == b'(' {
|
||||
answer.push(b'o');
|
||||
} else {
|
||||
answer.push(b'a');
|
||||
answer.push(b'l');
|
||||
}
|
||||
}
|
||||
pre = ch;
|
||||
}
|
||||
String::from_utf8(answer).unwrap()
|
||||
// command.replace("(al)", "al").replace("()", "o")
|
||||
}
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod test {
|
||||
use crate::Solution;
|
||||
|
||||
#[test]
|
||||
fn test_q1678() {
|
||||
assert_eq!(Solution::interpret("G()(al)".to_owned()), "Goal".to_owned());
|
||||
}
|
||||
}
|
|
@ -7,157 +7,6 @@ use std::rc::Rc;
|
|||
use std::ops::Add;
|
||||
|
||||
impl Solution {
|
||||
/// 面试题 16.01.交换数字
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/swap-numbers-lcci/)
|
||||
pub fn swap_numbers(mut numbers: Vec<i32>) -> Vec<i32> {
|
||||
numbers[0] ^= numbers[1];
|
||||
numbers[1] ^= numbers[0];
|
||||
numbers[0] ^= numbers[1];
|
||||
numbers
|
||||
}
|
||||
|
||||
/// 1404.将二进制表示减到 1 的步骤数
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/number-of-steps-to-reduce-a-number-in-binary-representation-to-one/)
|
||||
pub fn num_steps(s: String) -> i32 {
|
||||
let mut result = 0;
|
||||
let mut meet1 = false;
|
||||
|
||||
for t in s.chars().rev().enumerate() {
|
||||
if t.1 == '0' {
|
||||
result += if meet1 { 2 } else { 1 }
|
||||
} else {
|
||||
if !meet1 {
|
||||
if t.0 != (s.len() - 1) {
|
||||
result += 2;
|
||||
}
|
||||
meet1 = true;
|
||||
} else {
|
||||
result += 1;
|
||||
}
|
||||
}
|
||||
}
|
||||
result
|
||||
}
|
||||
|
||||
/// 面试题 08.03.魔术索引
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/magic-index-lcci/)
|
||||
///
|
||||
/// 循环遍历
|
||||
pub fn find_magic_index(nums: Vec<i32>) -> i32 {
|
||||
let mut idx = -1;
|
||||
for i in nums.iter().enumerate() {
|
||||
if *i.1 as usize == i.0 {
|
||||
return i.0 as i32;
|
||||
}
|
||||
}
|
||||
idx
|
||||
}
|
||||
|
||||
/// 面试题 16.07.最大数值
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/maximum-lcci/)
|
||||
///
|
||||
/// # 方法1
|
||||
/// 内置函数
|
||||
/// ```rust
|
||||
/// pub fn maximum(a: i32, b: i32) -> i32 {
|
||||
/// std::cmp::max(a, b)
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法2
|
||||
/// 数学公式 max(a, b) = (|a - b| + a + b) / 2。使用64位计算,避免溢出。实现见源码。
|
||||
pub fn maximum(a: i32, b: i32) -> i32 {
|
||||
((a as i64 - b as i64).abs() + a as i64 + b as i64 >> 1) as i32
|
||||
}
|
||||
|
||||
/// 面试题 05.01.插入
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/insert-into-bits-lcci/)
|
||||
///
|
||||
/// i到j位置0,或运算设置位
|
||||
pub fn insert_bits(n: i32, m: i32, i: i32, j: i32) -> i32 {
|
||||
n & !(((1 << (j - i + 1)) - 1) << i) | (m << i)
|
||||
}
|
||||
|
||||
/// 1480.一维数组的动态和
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/running-sum-of-1d-array/)
|
||||
pub fn running_sum(mut nums: Vec<i32>) -> Vec<i32> {
|
||||
nums.iter().scan(0, |s, i| {
|
||||
*s += *i;
|
||||
Some(*s)
|
||||
}).collect()
|
||||
}
|
||||
|
||||
/// 1281.整数的各位积和之差
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/subtract-the-product-and-sum-of-digits-of-an-integer/)
|
||||
pub fn subtract_product_and_sum(mut n: i32) -> i32 {
|
||||
let mut add = 0;
|
||||
let mut mul = 1;
|
||||
while n > 0 {
|
||||
let digit = n % 10;
|
||||
n /= 10;
|
||||
add += digit;
|
||||
mul *= digit;
|
||||
}
|
||||
mul - add
|
||||
}
|
||||
|
||||
/// 1295.统计位数为偶数的数字
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/find-numbers-with-even-number-of-digits/)
|
||||
///
|
||||
/// # 方法1 字符串长度
|
||||
/// ```rust
|
||||
/// pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
/// nums.iter().fold(0, |mut acc, x| {
|
||||
/// acc += (1usize - (x.to_string().len() & 1usize)) as i32;
|
||||
/// acc
|
||||
/// })
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法2 取以10为底的对数
|
||||
/// ```rust
|
||||
/// pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
/// nums.iter().map(|&x| { ((x as f32).log10()) as i32 & 1 }).sum()
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法3 除10
|
||||
/// ```rust
|
||||
/// pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
/// let mut res = 0;
|
||||
/// for mut num in nums {
|
||||
/// let mut b = 0;
|
||||
/// while num > 9 {
|
||||
/// num /= 10;
|
||||
/// b += 1;
|
||||
/// }
|
||||
/// res += b & 1;
|
||||
/// }
|
||||
/// res
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法4 暴力
|
||||
pub fn find_numbers(nums: Vec<i32>) -> i32 {
|
||||
let mut ret = 0i32;
|
||||
for n in nums {
|
||||
ret += match n {
|
||||
10..=99 | 1000..=9999 | 100000 => 1,
|
||||
_ => 0
|
||||
}
|
||||
}
|
||||
ret
|
||||
/*nums.iter().fold(0i32, |acc, &x| acc + match x {
|
||||
10..=99 | 1000..=9999 | 100000 => 1,
|
||||
_ => 0
|
||||
})*/
|
||||
//nums.iter().filter(|&&num| (10 <= num && num < 100) || (1000 <= num && num < 10000) || num == 100000).count() as i32
|
||||
}
|
||||
|
||||
/// 51.N皇后
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/n-queens/)
|
||||
|
@ -247,59 +96,6 @@ impl Solution {
|
|||
sell2
|
||||
}
|
||||
|
||||
/// 1342.将数字变成 0 的操作次数
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/number-of-steps-to-reduce-a-number-to-zero/)
|
||||
///
|
||||
/// # 方法1 递归
|
||||
/// ```rust
|
||||
/// pub fn number_of_steps (num: i32) -> i32 {
|
||||
/// if num > 1 {
|
||||
/// 1 + (num & 1) + number_of_steps(num >> 1)
|
||||
/// } else {
|
||||
/// num
|
||||
/// }
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法2 循环
|
||||
pub fn number_of_steps(mut num: i32) -> i32 {
|
||||
let mut res = 0;
|
||||
while num > 1 {
|
||||
res += 1 + (num & 1);
|
||||
num >>= 1;
|
||||
}
|
||||
res + num
|
||||
}
|
||||
|
||||
/// 1678.设计 Goal 解析器
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/goal-parser-interpretation/)
|
||||
///
|
||||
/// # 方法1 replase
|
||||
/// ```rust
|
||||
/// pub fn interpret(command: String) -> String {
|
||||
/// command.replace("(al)", "al").replace("()", "o")
|
||||
/// }
|
||||
/// ```
|
||||
/// # 方法2 遍历
|
||||
pub fn interpret(command: String) -> String {
|
||||
let mut answer = String::new();
|
||||
let mut pre = '\0';
|
||||
for ch in command.chars() {
|
||||
if ch == 'G' {
|
||||
answer.push(ch);
|
||||
} else if ch == ')' {
|
||||
if pre == '(' {
|
||||
answer.push('o');
|
||||
} else {
|
||||
answer.push_str("al");
|
||||
}
|
||||
}
|
||||
pre = ch;
|
||||
}
|
||||
answer
|
||||
}
|
||||
|
||||
/// LCP 06.拿硬币
|
||||
///
|
||||
/// [原题链接](https://leetcode-cn.com/problems/na-ying-bi/)
|
||||
|
@ -1062,12 +858,4 @@ mod tests {
|
|||
//println!("{}", ret);
|
||||
assert_eq!(ret, 20);
|
||||
}
|
||||
|
||||
#[test]
|
||||
fn test_find_numbers() {
|
||||
let i = vec![12, 345, 2, 6, 7896];
|
||||
let n = Solution::find_numbers(i);
|
||||
//println!("{}", n);
|
||||
assert_eq!(n, 2);
|
||||
}
|
||||
}
|
Loading…
Reference in New Issue